In this article, we are going to learn “How to create Youtube Search Engine using jQuery“. It is a easy jQuery script which help you to create Youtube Search Engine. you can use this in your website to search video on youtube which is publically available. It is very simple and easy to configure jQuery Step by Step.
Now, Let’s Start to make Youtube Search Engine with jQuery:
Step 1:
First of all you need to create google API key for this Project.
Click here to create Project for API key.
Select a project
Create new Project
Enter Project name
Select your created project and click on it
Search Youtube Data API and Enable Youtube Data API v3
Now Generate API key
Create credentials for Project
Finally we got get API key.
Step 2:
Create a html file and include below jQuery, Bootstrap, FancyBox and some script under <head> tag.
2 3 4 5 6 7 8 9 |
<script src="https://use.fontawesome.com/567fc88304.js"></script> <script src="http://cdnjs.cloudflare.com/ajax/libs/jquery/2.1.3/jquery.min.js"></script> <script src="http://cdnjs.cloudflare.com/ajax/libs/fancybox/2.1.5/jquery.fancybox.pack.js"></script> <script src="script.js"></script> <link rel="stylesheet" href="http://fancyapps.com/fancybox/source/jquery.fancybox.css?v=2.1.5"> <link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.6/css/bootstrap.min.css"> |
Step 3: Copy below HTML search form and paste after <body> tag.
2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 |
<div class="content"> <h2>YouTube Search Engine</h2> <div id="custom-search-input"> <div class="input-group col-md-12"> <form id="search-form" name="search-form" onsubmit="return searchYoutubevideo();"> <input type="text" class="form-control" placeholder="Search...." id="search" /> <span class="input-group-btn"> <button class="btn btn-info btn-lg" type="submit" id="findNow"> <i class="glyphicon glyphicon-search"></i> </button> </span> </form> </div> </div> <div id="results"></div> <div id="buttons"></div> </div> |
Step 4: Add below css code under <head> tag to make output look good.
2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 |
<style> #custom-search-input{ padding: 3px; border: solid 1px #E4E4E4; border-radius: 6px; background-color: #fff; } #custom-search-input input{ border: 0; box-shadow: none; width: 93%; } #custom-search-input button{ margin: 2px 0 0 0; background: none; box-shadow: none; border: 0; color: #666666; padding: 0 8px 0 10px; border-left: solid 1px #ccc; } #custom-search-input button:hover{ border: 0; box-shadow: none; border-left: solid 1px #ccc; } #custom-search-input .glyphicon-search{ font-size: 23px; } article { margin: 10px 0; background: rgba(0,0,0,0.7); padding: 4px 20px; } .video-wrapper { position: fixed; top: 0; right: 0; bottom: 0; left: 0; overflow: hidden; z-index:-1; } .video-wrapper video { position: fixed; top: 0; right: 0; min-width: 100%; min-height: 100%; width: auto; height: auto; } .content { position: relative; margin: 60px auto; width: 80%; max-width: 640px; z-index: 1; } .clearfix{ border-bottom: 1px dotted #ccc; margin-bottom: 5px; } </style> |
Step 5: Now add jQuery code after HTML form code and replace your API Key with this.
2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 167 168 169 170 171 172 173 174 175 176 177 178 179 180 181 182 183 184 185 186 187 188 189 190 191 192 193 194 195 196 197 198 |
<script> var gapikey = 'ADD_YOUR_API_KEY_HERE'; $(function() { // call fancybox pluggin $(".fancyboxIframe").fancybox({ maxWidth : 900, maxHeight : 600, fitToView : false, width : '90%', height : '90%', autoSize : false, closeClick : false, openEffect : 'none', closeEffect : 'none', iframe: { scrolling : 'auto', preload : true } }); $('#search-form').submit( function(e) { e.preventDefault(); }); }); function searchYoutubevideo() { $('#results').html('<i class="fa fa-spinner fa-pulse fa-3x fa-fw"></i><span class="sr-only">Loading...</span>'); $('#buttons').html(''); // get form input q = $('#search').val(); // run get request on API $.get( "https://www.googleapis.com/youtube/v3/search", { part: 'snippet, id', q: q, type: 'video', key: gapikey }, function(data) { var nextPageToken = data.nextPageToken; var prevPageToken = data.prevPageToken; // Log data console.log(data); $('#results').html(''); // hide loading $.each(data.items, function(i, item) { // Get Output var output = getOutput(item); // display results $('#results').append(output); }); var buttons = getButtons(prevPageToken, nextPageToken); // Display buttons $('#buttons').append(buttons); }); } // Next page function function nextPage() { var token = $('#next-button').data('token'); var q = $('#next-button').data('query'); // clear $('#results').html('<i class="fa fa-spinner fa-pulse fa-3x fa-fw"></i><span class="sr-only">Loading...</span>'); $('#buttons').html(''); // get form input q = $('#search').val(); // run get request on API $.get( "https://www.googleapis.com/youtube/v3/search", { part: 'snippet, id', q: q, pageToken: token, type: 'video', key: gapikey }, function(data) { var nextPageToken = data.nextPageToken; var prevPageToken = data.prevPageToken; // Log data console.log(data); $('#results').html(''); $.each(data.items, function(i, item) { // Get Output var output = getOutput(item); // display results $('#results').append(output); }); var buttons = getButtons(prevPageToken, nextPageToken); // Display buttons $('#buttons').append(buttons); }); } // Previous page function function prevPage() { var token = $('#prev-button').data('token'); var q = $('#prev-button').data('query'); // clear $('#results').html('<i class="fa fa-spinner fa-pulse fa-3x fa-fw"></i><span class="sr-only">Loading...</span>'); $('#buttons').html(''); // get form input q = $('#search').val(); // this probably shouldn't be created as a global // run get request on API $.get( "https://www.googleapis.com/youtube/v3/search", { part: 'snippet, id', q: q, pageToken: token, type: 'video', key: gapikey }, function(data) { var nextPageToken = data.nextPageToken; var prevPageToken = data.prevPageToken; // Log data console.log(data); $('#results').html(''); $.each(data.items, function(i, item) { // Get Output var output = getOutput(item); // display results $('#results').append(output); }); var buttons = getButtons(prevPageToken, nextPageToken); // Display buttons $('#buttons').append(buttons); }); } // Build output function getOutput(item) { var videoID = item.id.videoId; var title = item.snippet.title; var description = item.snippet.description; var thumb = item.snippet.thumbnails.high.url; var channelTitle = item.snippet.channelTitle; var videoDate = item.snippet.publishedAt; // Build output string var output = '<div class="col-md-6">' + '<img src="' + thumb + '" class="img-responsive thumbnail" >' + '</div>' + '<div class="input-group col-md-6">' + '<h3><a data-fancybox-type="iframe" class="fancyboxIframe" href="http://youtube.com/embed/' + videoID + '?rel=0">' + title + '</a></h3>' + '<small>By <span class="channel">' + channelTitle + '</span> on ' + videoDate + '</small>' + '<p>' + description + '</p>' + '</div>' + '<div class="clearfix"></div>'; return output; } function getButtons(prevPageToken, nextPageToken) { if(!prevPageToken) { var btnoutput = '<ul class="pagination">' + '<li><a href="javascript:;" id="next-button" class="paging-button" data-token="' + nextPageToken + '" data-query="' + q + '"' + 'onclick = "nextPage();">Next »</a></li>' + '</ul>'; } else { var btnoutput = '<ul class="pagination">' + '<li><a href="javascript:;" id="prev-button" class="paging-button" data-token="' + prevPageToken + '" data-query="' + q + '"' + 'onclick = "prevPage();">« Previous</a></li>' + '<li><a href="javascript:;" id="next-button" class="paging-button" data-token="' + nextPageToken + '" data-query="' + q + '"' + 'onclick = "nextPage();">Next »</a></li>' + '</ul>'; } return btnoutput; } </script> |
Above jQuery is used to request API’s and fetch data from youtube server using you API Key.
searchYoutubevideo() : This function search all video from youtube server for given API key
nextPage() and prevPage(): show next page and previous page of current result
getOutput(item): This function create output data on given values
getButtons(prevPageToken, nextPageToken): Create button for next and previous step.
I hope you will like this tutorial and please share your feedback in comments box and if you have any please comment below.
[sociallocker]
[/sociallocker]
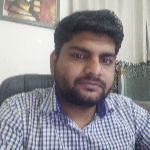
Pradeep Maurya is the Professional Web Developer & Designer and the Founder of “Tutorials website”. He lives in Delhi and loves to be a self-dependent person. As an owner, he is trying his best to improve this platform day by day. His passion, dedication and quick decision making ability to stand apart from others. He’s an avid blogger and writes on the publications like Dzone, e27.co